Deep Dive into TFLite On-Device Training
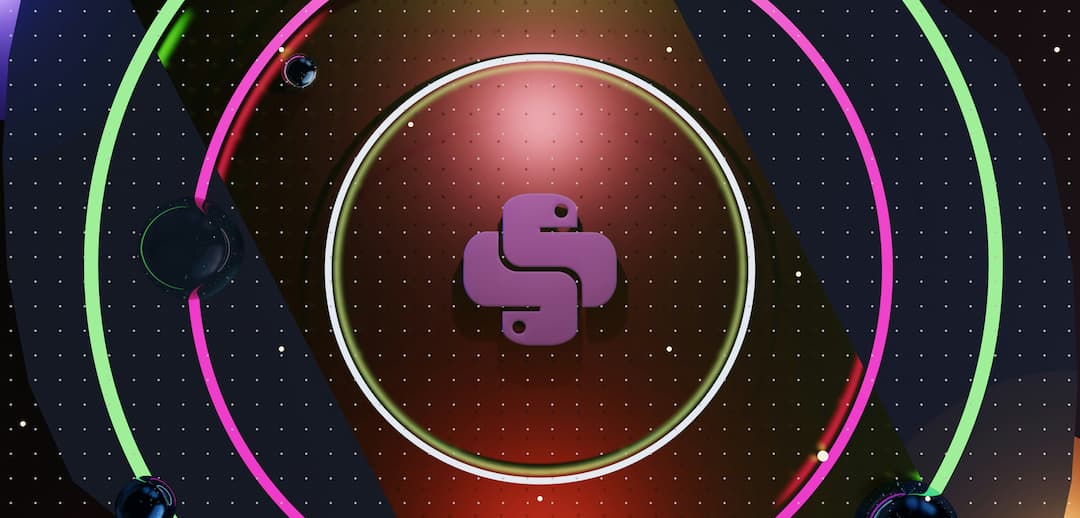
Introduction
TensorFlow has revolutionized the field of machine learning by providing powerful tools for developers. Now, with on-device machine learning, TensorFlow is pushing the boundaries even further. This exciting capability allows machine learning models to run directly on user devices, unlocking new possibilities for performance, privacy, and personalized experiences. Let's delve into the world of TensorFlow Lite on-device training!
What is TFLite?
TensorFlow Lite (TFLite) is an open-source framework developed by Google. Its primary function is to efficiently run machine learning models on mobile devices and edge devices. Edge devices encompass a wide range of gadgets with limited processing power, including smartphones, embedded systems, and even tiny microcontrollers.
TFLite achieves this by streamlining models created with TensorFlow. It converts them into a format that can execute efficiently on these resource-constrained devices. This conversion process often involves reducing the model's size and complexity while striving to maintain its accuracy.
Key Benefits of TFLite
- On-device execution: TFLite models run directly on the device, eliminating the need to send data back and forth to a server. This translates to faster performance, improved privacy (data stays on the device), and functionality even without an internet connection.
- Optimized for edge devices: TFLite is specifically designed to be small and efficient, making it ideal for devices with limited processing power and memory.
- Multi-platform support: TFLite models can run on a variety of platforms, including Android, iOS, embedded Linux, and even microcontrollers.
How to Convert a Model to TFLite
python
import tensorflow as tf
# Load your TensorFlow model
model = tf.keras.models.load_model('your_model.h5')
# Convert to TensorFlow Lite format
converter = tf.lite.TFLiteConverter.from_keras_model(model)
tflite_model = converter.convert()
# Save the optimized model
with open('model.tflite', 'wb') as f:
f.write(tflite_model)
TFLite On-Device Training
TensorFlow Lite (TFLite) takes machine learning (ML) a step further by enabling on-device training. This empowers you to refine pre-trained models directly on the devices they run on, using new, user-generated data. This opens doors for exciting applications that personalize experiences, adapt to specific environments, and prioritize user privacy.
TFLite On-Device Training Involves a Cyclical Interplay Between Four Key Functions:
- Restore: This function retrieves the previously saved model state, essentially reloading the model's weights (parameters) learned during past training sessions. It acts as a knowledge retrieval mechanism, ensuring the model builds upon prior learning.
- Train: The heart of on-device training, this function takes a batch of new data as input. It feeds this data through the model, calculates the discrepancy between predictions and actual labels (loss), and utilizes an optimizer to adjust the weights in a way that minimizes the loss. This iterative process refines the model's ability to perform the specific task.
- Save: This function captures the model's current state, including the updated weights obtained after a training session. Saving the model is crucial for preserving learning progress and enabling continued training or model utilization for inference later.
- Infer: This function empowers the model to make predictions on unseen data. Once training is complete, the infer function takes new data as input and leverages the model's learned patterns to generate predictions. This allows the model to fulfill its intended purpose, such as image classification or anomaly detection directly on the device.
Synergy for Continuous Learning
These functions work in a harmonious loop:
- Restore the model's knowledge from the previous training session.
- Train the model on a new batch of data to further refine its performance.
- Save the updated model state to preserve progress.
- Use the infer function to make predictions on new data, demonstrating the model's learning.
By effectively orchestrating these functions, you can create TFLite models that continuously learn and adapt on the device, unlocking the potential of on-device machine learning.
Core of On-Device Training
- Pre-Trained Foundation: The journey begins with a model trained on a vast dataset for a particular task (e.g., image classification). This model serves as a solid base.
- Fresh Data Injection: New data, often collected directly on the device, is used to further train the model. This data can be from user interactions or sensor readings, allowing for personalization or adaptation.
- On-Device Refinement: Leveraging the device's processing power, the pre-trained model is fine-tuned with the new data. This potentially improves the model's performance for the specific user or environment.
Capabilities and Considerations
- Ideal Applications: On-device training shines in scenarios where new data is constantly generated and can benefit the model. Imagine anomaly detection in sensors that improves over time, or language models that personalize text prediction based on user vocabulary.
- Resource Constraints: Remember, edge devices like smartphones have limitations in processing power and memory. Training very complex models or massive datasets might not be practical.
Saving Tflite model with defined Signatures
Step 1: Defining the Base TFLite Model
The foundation of our TFLite model starts with a base class BaseTFLiteModel
. This class provides essential methods and attributes that any TFLite-compatible model should implement. Here's a breakdown of its components:
python
class BaseTFLiteModel(tf.Module):
"""Base TFLite model class to inherit from.
Usage: Inherit from this class and annotate with @tflite_model_class.
Attributes to override:
- X_SHAPE: Shape of the input to the model.
- Y_SHAPE: Shape of the output from the model.
- model: tf.keras.Model instance.
Provides default implementations of train, infer, parameters, restore.
These methods are not annotated with @tf.function; they are supposed to be
converted by @tflite_model_class.
"""
X_SHAPE: list[int]
Y_SHAPE: list[int]
model: tf.keras.Model
def train(self, x, y):
return self.model.train_step((x, y))
def infer(self, x):
return {"logits": self.model(x)}
def parameters(self):
return {
f"a{index}": weight
for index, weight in enumerate(self.model.weights)
}
def restore(self, **parameters):
for index, weight in enumerate(self.model.weights):
parameter = parameters[f"a{index}"]
weight.assign(parameter)
assert self.parameters is not None
return self.parameters()
Step 2: Converting to TFLite Model Class
To make the model TFLite compatible, we use the tflite_model_class
decorator. This decorator converts methods using @tf.function
with appropriate input_signature
based on X_SHAPE
and Y_SHAPE
.
python
def tflite_model_class(cls):
"""Convert cls that inherits from BaseTFLiteModel to a TFLite model class."""
cls.x_spec = tf.TensorSpec([None] + cls.X_SHAPE, tf.float32)
cls.y_spec = tf.TensorSpec([None] + cls.Y_SHAPE, tf.float32)
cls.train = tf.function(cls.train, input_signature=[cls.x_spec, cls.y_spec])
cls.infer = tf.function(cls.infer, input_signature=[cls.x_spec])
cls.parameters = tf.function(cls.parameters, input_signature=[])
cls.restore = tf.function(cls.restore)
return cls
Step 3: Saving the TFLite Model
Once the TensorFlow model is annotated and prepared with @tflite_model_class
, it can be saved as a TFLite model using tf.saved_model.save
.
python
def save_model(model, saved_model_dir):
"""Saves a TensorFlow model to the specified directory."""
signatures = {
"train": model.train.get_concrete_function(),
"infer": model.infer.get_concrete_function(),
"parameters": model.parameters.get_concrete_function(),
"restore": model.restore.get_concrete_function(),
}
tf.saved_model.save(model, saved_model_dir, signatures=signatures)
Step 4: Converting to TFLite Format
Finally, convert the saved TensorFlow model to a TFLite model format using tf.lite.TFLiteConverter
.
python
def convert_saved_model(saved_model_dir):
converter = tf.lite.TFLiteConverter.from_saved_model(saved_model_dir)
tflite_model = converter.convert()
return tflite_model
Conclusion
In this guide, we've covered the essential steps to convert a TensorFlow model into a TFLite model suitable for deployment on mobile and embedded platforms. By leveraging TensorFlow's capabilities, including tf.function
for method conversion and tf.lite.TFLiteConverter
for format conversion, you can seamlessly optimize and deploy machine learning models to edge devices.
By following these steps, you can effectively bridge the gap between training complex machine learning models in TensorFlow and deploying them in resource-constrained environments using TensorFlow Lite.